Here we present C notes in PDF or C programming language eBook for computer programmers and software developers. It’s created/compiled by GoalKicker and free to download. C is a great programming language and concepts and hands-on expertise in it is a great plus for programmers and developers.
C language eBook | C notes in PDF | free download
The pdf notes on C is embedded below for your reference and study.
What you get in the ebook – Content Details
Chapter 1: Getting started with C Language …………………………………………………………………………………….. 2
Section 1.1: Hello World ………………………………………………………………………………………………………………………………. 2
Section 1.2: Original “Hello, World!” in K&R C ……………………………………………………………………………………………….. 4
Chapter 2: Comments ………………………………………………………………………………………………………………………………. 6
Section 2.1: Commenting using the preprocessor ………………………………………………………………………………………… 6
Section 2.2: /* */ delimited comments ………………………………………………………………………………………………………… 6
Section 2.3: // delimited comments ……………………………………………………………………………………………………………. 7
Section 2.4: Possible pitfall due to trigraphs ……………………………………………………………………………………………….. 7
Chapter 3: Data Types …………………………………………………………………………………………………………………………….. 9
Section 3.1: Interpreting Declarations ………………………………………………………………………………………………………….. 9
Section 3.2: Fixed Width Integer Types (since C99) ……………………………………………………………………………………. 11
Section 3.3: Integer types and constants …………………………………………………………………………………………………… 11
Section 3.4: Floating Point Constants ………………………………………………………………………………………………………… 12
Section 3.5: String Literals ………………………………………………………………………………………………………………………… 13
Chapter 4: Operators …………………………………………………………………………………………………………………………….. 14
Section 4.1: Relational Operators ………………………………………………………………………………………………………………. 14
Section 4.2: Conditional Operator/Ternary Operator ………………………………………………………………………………… 15
Section 4.3: Bitwise Operators ………………………………………………………………………………………………………………….. 16
Section 4.4: Short circuit behavior of logical operators ……………………………………………………………………………… 18
Section 4.5: Comma Operator ………………………………………………………………………………………………………………….. 19
Section 4.6: Arithmetic Operators ……………………………………………………………………………………………………………… 19
Section 4.7: Access Operators ………………………………………………………………………………………………………………….. 22
Section 4.8: sizeof Operator ……………………………………………………………………………………………………………………… 24
Section 4.9: Cast Operator ……………………………………………………………………………………………………………………….. 24
Section 4.10: Function Call Operator …………………………………………………………………………………………………………. 24
Section 4.11: Increment / Decrement …………………………………………………………………………………………………………. 25
Section 4.12: Assignment Operators ………………………………………………………………………………………………………….. 25
Section 4.13: Logical Operators …………………………………………………………………………………………………………………. 26
Section 4.14: Pointer Arithmetic …………………………………………………………………………………………………………………. 27
Section 4.15: _Alignof ……………………………………………………………………………………………………………………………….. 28
Chapter 5: Boolean …………………………………………………………………………………………………………………………………. 30
Section 5.1: Using stdbool.h ………………………………………………………………………………………………………………………. 30
Section 5.2: Using #define ………………………………………………………………………………………………………………………… 30
Section 5.3: Using the Intrinsic (built-in) Type _Bool ………………………………………………………………………………….. 31
Section 5.4: Integers and pointers in Boolean expressions ………………………………………………………………………… 31
Section 5.5: Defining a bool type using typedef ………………………………………………………………………………………… 32
Chapter 6: Strings ……………………………………………………………………………………………………………………………………. 33
Section 6.1: Tokenisation: strtok(), strtok_r() and strtok_s() ………………………………………………………………………. 33
Section 6.2: String literals …………………………………………………………………………………………………………………………. 35
Section 6.3: Calculate the Length: strlen() …………………………………………………………………………………………………. 36
Section 6.4: Basic introduction to strings …………………………………………………………………………………………………… 37
Section 6.5: Copying strings ……………………………………………………………………………………………………………………… 37
Section 6.6: Iterating Over the Characters in a String ………………………………………………………………………………… 40
Section 6.7: Creating Arrays of Strings ……………………………………………………………………………………………………… 41
Section 6.8: Convert Strings to Number: atoi(), atof() (dangerous, don’t use them) ……………………………………. 41
Section 6.9: string formatted data read/write …………………………………………………………………………………………… 42
Section 6.10: Find first/last occurrence of a specific character: strchr(), strrchr() ……………………………………….. 43
Section 6.11: Copy and Concatenation: strcpy(), strcat() ……………………………………………………………………………. 44
Section 6.12: Comparsion: strcmp(), strncmp(), strcasecmp(), strncasecmp() ……………………………………………. 45
Section 6.13: Safely convert Strings to Number: strtoX functions ……………………………………………………………….. 47
Section 6.14: strspn and strcspn ………………………………………………………………………………………………………………… 48
Chapter 7: Literals for numbers, characters and strings ……………………………………………………………. 50
Section 7.1: Floating point literals ………………………………………………………………………………………………………………. 50
Section 7.2: String literals …………………………………………………………………………………………………………………………. 50
Section 7.3: Character literals …………………………………………………………………………………………………………………… 50
Section 7.4: Integer literals ……………………………………………………………………………………………………………………….. 51
Chapter 8: Compound Literals …………………………………………………………………………………………………………….. 53
Section 8.1: Definition/Initialisation of Compound Literals ………………………………………………………………………….. 53
Chapter 9: Bit-fields ……………………………………………………………………………………………………………………………….. 55
Section 9.1: Bit-fields …………………………………………………………………………………………………………………………………. 55
Section 9.2: Using bit-fields as small integers ……………………………………………………………………………………………. 56
Section 9.3: Bit-field alignment …………………………………………………………………………………………………………………. 56
Section 9.4: Don’ts for bit-fields ………………………………………………………………………………………………………………… 57
Section 9.5: When are bit-fields useful? …………………………………………………………………………………………………….. 58
Chapter 10: Arrays …………………………………………………………………………………………………………………………………… 60
Section 10.1: Declaring and initializing an array …………………………………………………………………………………………. 60
Section 10.2: Iterating through an array eciently and row-major order …………………………………………………… 61
Section 10.3: Array length …………………………………………………………………………………………………………………………. 62
Section 10.4: Passing multidimensional arrays to a function ……………………………………………………………………… 63
Section 10.5: Multi-dimensional arrays ………………………………………………………………………………………………………. 64
Section 10.6: Define array and access array element ………………………………………………………………………………… 67
Section 10.7: Clearing array contents (zeroing) …………………………………………………………………………………………. 67
Section 10.8: Setting values in arrays ………………………………………………………………………………………………………… 68
Section 10.9: Allocate and zero-initialize an array with user defined size ……………………………………………………. 68
Section 10.10: Iterating through an array using pointers ……………………………………………………………………………. 69
Chapter 11: Linked lists …………………………………………………………………………………………………………………………… 71
Section 11.1: A doubly linked list …………………………………………………………………………………………………………………. 71
Section 11.2: Reversing a linked list ……………………………………………………………………………………………………………. 73
Section 11.3: Inserting a node at the nth position ……………………………………………………………………………………….. 75
Section 11.4: Inserting a node at the beginning of a singly linked list ………………………………………………………….. 76
Chapter 12: Enumerations …………………………………………………………………………………………………………………….. 79
Section 12.1: Simple Enumeration ………………………………………………………………………………………………………………. 79
Section 12.2: enumeration constant without typename ……………………………………………………………………………… 80
Section 12.3: Enumeration with duplicate value …………………………………………………………………………………………. 80
Section 12.4: Typedef enum ……………………………………………………………………………………………………………………… 81
Chapter 13: Structs ………………………………………………………………………………………………………………………………….. 83
Section 13.1: Flexible Array Members …………………………………………………………………………………………………………. 83
Section 13.2: Typedef Structs ……………………………………………………………………………………………………………………. 85
Section 13.3: Pointers to structs …………………………………………………………………………………………………………………. 86
Section 13.4: Passing structs to functions ………………………………………………………………………………………………….. 88
Section 13.5: Object-based programming using structs …………………………………………………………………………….. 89
Section 13.6: Simple data structures ………………………………………………………………………………………………………….. 91
Chapter 14: Standard Math ………………………………………………………………………………………………………………….. 93
Section 14.1: Power functions – pow(), powf(), powl() …………………………………………………………………………………. 93
Section 14.2: Double precision floating-point remainder: fmod() ……………………………………………………………….. 94
Section 14.3: Single precision and long double precision floating-point remainder: fmodf(), fmodl() …………… 94
Chapter 15: Iteration Statements/Loops: for, while, do-while …………………………………………………… 96
Section 15.1: For loop ………………………………………………………………………………………………………………………………… 96
Section 15.2: Loop Unrolling and Du’s Device ………………………………………………………………………………………….. 96
Section 15.3: While loop …………………………………………………………………………………………………………………………….. 97
Section 15.4: Do-While loop ………………………………………………………………………………………………………………………. 97
Section 15.5: Structure and flow of control in a for loop …………………………………………………………………………….. 98
Section 15.6: Infinite Loops ………………………………………………………………………………………………………………………… 99
Chapter 16: Selection Statements …………………………………………………………………………………………………….. 100
Section 16.1: if () Statements ……………………………………………………………………………………………………………………. 100
Section 16.2: Nested if()…else VS if()..else Ladder …………………………………………………………………………………….. 100
Section 16.3: switch () Statements …………………………………………………………………………………………………………… 102
Section 16.4: if () … else statements and syntax ……………………………………………………………………………………….. 104
Section 16.5: if()…else Ladder Chaining two or more if () … else statements ………………………………………………. 104
Chapter 17: Initialization ………………………………………………………………………………………………………………………. 105
Section 17.1: Initialization of Variables in C ……………………………………………………………………………………………….. 105
Section 17.2: Using designated initializers ………………………………………………………………………………………………… 106
Section 17.3: Initializing structures and arrays of structures …………………………………………………………………….. 108
Chapter 18: Declaration vs Definition ……………………………………………………………………………………………… 110
Section 18.1: Understanding Declaration and Definition …………………………………………………………………………… 110
Chapter 19: Command-line arguments …………………………………………………………………………………………… 111
Section 19.1: Print the arguments to a program and convert to integer values …………………………………………. 111
Section 19.2: Printing the command line arguments ………………………………………………………………………………… 111
Section 19.3: Using GNU getopt tools ………………………………………………………………………………………………………. 112
Chapter 20: Files and I/O streams …………………………………………………………………………………………………… 115
Section 20.1: Open and write to file …………………………………………………………………………………………………………. 115
Section 20.2: Run process ………………………………………………………………………………………………………………………. 116
Section 20.3: fprintf ………………………………………………………………………………………………………………………………… 116
Section 20.4: Get lines from a file using getline() …………………………………………………………………………………….. 116
Section 20.5: fscanf() ……………………………………………………………………………………………………………………………… 120
Section 20.6: Read lines from a file …………………………………………………………………………………………………………. 121
Section 20.7: Open and write to a binary file …………………………………………………………………………………………… 122
Chapter 21: Formatted Input/Output ………………………………………………………………………………………………. 124
Section 21.1: Conversion Specifiers for printing ………………………………………………………………………………………… 124
Section 21.2: The printf() Function …………………………………………………………………………………………………………… 125
Section 21.3: Printing format flags …………………………………………………………………………………………………………… 125
Section 21.4: Printing the Value of a Pointer to an Object ………………………………………………………………………… 126
Section 21.5: Printing the Dierence of the Values of two Pointers to an Object ……………………………………….. 127
Section 21.6: Length modifiers ………………………………………………………………………………………………………………… 128
Chapter 22: Pointers ……………………………………………………………………………………………………………………………… 129
Section 22.1: Introduction ……………………………………………………………………………………………………………………….. 129
Section 22.2: Common errors …………………………………………………………………………………………………………………. 131
Section 22.3: Dereferencing a Pointer …………………………………………………………………………………………………….. 134
Section 22.4: Dereferencing a Pointer to a struct …………………………………………………………………………………….. 134
Section 22.5: Const Pointers ……………………………………………………………………………………………………………………. 135
Section 22.6: Function pointers ……………………………………………………………………………………………………………….. 138
Section 22.7: Polymorphic behaviour with void pointers ………………………………………………………………………….. 139
Section 22.8: Address-of Operator ( & ) ………………………………………………………………………………………………….. 140
Section 22.9: Initializing Pointers …………………………………………………………………………………………………………….. 140
Section 22.10: Pointer to Pointer ……………………………………………………………………………………………………………… 141
Section 22.11: void* pointers as arguments and return values to standard functions ………………………………… 141
Section 22.12: Same Asterisk, Dierent Meanings ……………………………………………………………………………………. 142
Chapter 23: Sequence points ……………………………………………………………………………………………………………… 144
Section 23.1: Unsequenced expressions …………………………………………………………………………………………………… 144
Section 23.2: Sequenced expressions ……………………………………………………………………………………………………… 144
Section 23.3: Indeterminately sequenced expressions …………………………………………………………………………….. 145
Chapter 24: Function Pointers …………………………………………………………………………………………………………… 146
Section 24.1: Introduction ………………………………………………………………………………………………………………………… 146
Section 24.2: Returning Function Pointers from a Function ……………………………………………………………………… 146
Section 24.3: Best Practices ……………………………………………………………………………………………………………………. 147
Section 24.4: Assigning a Function Pointer ………………………………………………………………………………………………. 149
Section 24.5: Mnemonic for writing function pointers ………………………………………………………………………………. 149
Section 24.6: Basics ………………………………………………………………………………………………………………………………… 150
Chapter 25: Function Parameters …………………………………………………………………………………………………….. 152
Section 25.1: Parameters are passed by value ………………………………………………………………………………………… 152
Section 25.2: Passing in Arrays to Functions ……………………………………………………………………………………………. 152
Section 25.3: Order of function parameter execution ………………………………………………………………………………. 153
Section 25.4: Using pointer parameters to return multiple values ……………………………………………………………. 153
Section 25.5: Example of function returning struct containing values with error codes …………………………….. 154
Chapter 26: Pass 2D-arrays to functions ……………………………………………………………………………………….. 156
Section 26.1: Pass a 2D-array to a function …………………………………………………………………………………………….. 156
Section 26.2: Using flat arrays as 2D arrays ……………………………………………………………………………………………. 162
Chapter 27: Error handling …………………………………………………………………………………………………………………. 163
Section 27.1: errno ………………………………………………………………………………………………………………………………….. 163
Section 27.2: strerror ………………………………………………………………………………………………………………………………. 163
Section 27.3: perror ………………………………………………………………………………………………………………………………… 163
Chapter 28: Undefined behavior ………………………………………………………………………………………………………. 165
Section 28.1: Dereferencing a pointer to variable beyond its lifetime ……………………………………………………….. 165
Section 28.2: Copying overlapping memory ……………………………………………………………………………………………. 165
Section 28.3: Signed integer overflow ……………………………………………………………………………………………………… 166
Section 28.4: Use of an uninitialized variable …………………………………………………………………………………………… 167
Section 28.5: Data race ………………………………………………………………………………………………………………………….. 168
Section 28.6: Read value of pointer that was freed …………………………………………………………………………………. 169
Section 28.7: Using incorrect format specifier in printf …………………………………………………………………………….. 170
Section 28.8: Modify string literal ……………………………………………………………………………………………………………. 170
Section 28.9: Passing a null pointer to printf %s conversion …………………………………………………………………….. 170
Section 28.10: Modifying any object more than once between two sequence points ………………………………… 171
Section 28.11: Freeing memory twice ………………………………………………………………………………………………………. 172
Section 28.12: Bit shifting using negative counts or beyond the width of the type …………………………………….. 172
Section 28.13: Returning from a function that’s declared with _Noreturn
or noreturn
function specifier
……………………………………………………………………………………………………………………………………………………….. 173
Section 28.14: Accessing memory beyond allocated chunk ……………………………………………………………………… 174
Section 28.15: Modifying a const variable using a pointer ………………………………………………………………………… 174
Section 28.16: Reading an uninitialized object that is not backed by memory ………………………………………….. 175
Section 28.17: Addition or subtraction of pointer not properly bounded …………………………………………………… 175
Section 28.18: Dereferencing a null pointer ……………………………………………………………………………………………… 175
Section 28.19: Using ush on an input stream ………………………………………………………………………………………… 176
Section 28.20: Inconsistent linkage of identifiers ……………………………………………………………………………………… 176
Section 28.21: Missing return statement in value returning function …………………………………………………………. 177
Section 28.22: Division by zero ……………………………………………………………………………………………………………….. 177
Section 28.23: Conversion between pointer types produces incorrectly aligned result ……………………………… 178
Section 28.24: Modifying the string returned by getenv, strerror, and setlocale functions ………………………… 179
Chapter 29: Random Number Generation ……………………………………………………………………………………… 180
Section 29.1: Basic Random Number Generation …………………………………………………………………………………….. 180
Section 29.2: Permuted Congruential Generator ……………………………………………………………………………………… 180
Section 29.3: Xorshift Generation ……………………………………………………………………………………………………………. 181
Section 29.4: Restrict generation to a given range ………………………………………………………………………………….. 182
Chapter 30: Preprocessor and Macros ……………………………………………………………………………………………. 183
Section 30.1: Header Include Guards ……………………………………………………………………………………………………….. 183
Section 30.2: #if 0 to block out code sections ………………………………………………………………………………………….. 186
Section 30.3: Function-like macros ………………………………………………………………………………………………………….. 187
Section 30.4: Source file inclusion ……………………………………………………………………………………………………………. 188
Section 30.5: Conditional inclusion and conditional function signature modification ………………………………… 188
Section 30.6: __cplusplus for using C externals in C++ code compiled with C++ – name mangling …………… 190
Section 30.7: Token pasting ……………………………………………………………………………………………………………………. 191
Section 30.8: Predefined Macros …………………………………………………………………………………………………………….. 192
Section 30.9: Variadic arguments macro ………………………………………………………………………………………………… 193
Section 30.10: Macro Replacement …………………………………………………………………………………………………………. 194
Section 30.11: Error directive ……………………………………………………………………………………………………………………. 195
Section 30.12: FOREACH implementation ………………………………………………………………………………………………… 196
Chapter 31: Signal handling ………………………………………………………………………………………………………………… 199
Section 31.1: Signal Handling with “signal()” ……………………………………………………………………………………………… 199
Chapter 32: Variable arguments ………………………………………………………………………………………………………. 201
Section 32.1: Using an explicit count argument to determine the length of the va_list ……………………………… 201
Section 32.2: Using terminator values to determine the end of va_list …………………………………………………….. 202
Section 32.3: Implementing functions with a printf()
-like interface …………………………………………………………. 202
Section 32.4: Using a format string …………………………………………………………………………………………………………. 205
Chapter 33: Assertion …………………………………………………………………………………………………………………………… 207
Section 33.1: Simple Assertion …………………………………………………………………………………………………………………. 207
Section 33.2: Static Assertion ………………………………………………………………………………………………………………….. 207
Section 33.3: Assert Error Messages ……………………………………………………………………………………………………….. 208
Section 33.4: Assertion of Unreachable Code ………………………………………………………………………………………….. 209
Section 33.5: Precondition and Postcondition ………………………………………………………………………………………….. 209
Chapter 34: Generic selection ……………………………………………………………………………………………………………. 211
Section 34.1: Check whether a variable is of a certain qualified type ………………………………………………………… 211
Section 34.2: Generic selection based on multiple arguments ………………………………………………………………….. 211
Section 34.3: Type-generic printing macro ……………………………………………………………………………………………… 213
Chapter 35: X-macros ………………………………………………………………………………………………………………………….. 214
Section 35.1: Trivial use of X-macros for printfs ……………………………………………………………………………………….. 214
Section 35.2: Extension: Give the X macro as an argument ……………………………………………………………………… 214
Section 35.3: Enum Value and Identifier ………………………………………………………………………………………………….. 215
Section 35.4: Code generation ………………………………………………………………………………………………………………… 215
Chapter 36: Aliasing and eective type …………………………………………………………………………………………. 217
Section 36.1: Eective type ……………………………………………………………………………………………………………………… 217
Section 36.2: restrict qualification ……………………………………………………………………………………………………………. 217
Section 36.3: Changing bytes ………………………………………………………………………………………………………………….. 218
Section 36.4: Character types cannot be accessed through non-character types ……………………………………. 219
Section 36.5: Violating the strict aliasing rules …………………………………………………………………………………………. 220
Chapter 37: Compilation ……………………………………………………………………………………………………………………… 221
Section 37.1: The Compiler ………………………………………………………………………………………………………………………. 221
Section 37.2: File Types ………………………………………………………………………………………………………………………….. 222
Section 37.3: The Linker ………………………………………………………………………………………………………………………….. 222
Section 37.4: The Preprocessor ………………………………………………………………………………………………………………. 224
Section 37.5: The Translation Phases ………………………………………………………………………………………………………. 225
Chapter 38: Inline assembly ……………………………………………………………………………………………………………….. 227
Section 38.1: gcc Inline assembly in macros …………………………………………………………………………………………….. 227
Section 38.2: gcc Basic asm support ………………………………………………………………………………………………………. 227
Section 38.3: gcc Extended asm support …………………………………………………………………………………………………. 228
Chapter 39: Identifier Scope ………………………………………………………………………………………………………………. 229
Section 39.1: Function Prototype Scope …………………………………………………………………………………………………… 229
Section 39.2: Block Scope ……………………………………………………………………………………………………………………….. 230
Section 39.3: File Scope ………………………………………………………………………………………………………………………….. 230
Section 39.4: Function scope …………………………………………………………………………………………………………………… 231
Chapter 40: Implicit and Explicit Conversions ………………………………………………………………………………. 232
Section 40.1: Integer Conversions in Function Calls ………………………………………………………………………………….. 232
Section 40.2: Pointer Conversions in Function Calls …………………………………………………………………………………. 233
Chapter 41: Type Qualifiers ……………………………………………………………………………………………………………….. 235
Section 41.1: Volatile variables …………………………………………………………………………………………………………………. 235
Section 41.2: Unmodifiable (const) variables …………………………………………………………………………………………… 236
Chapter 42: Typedef ……………………………………………………………………………………………………………………………. 237
Section 42.1: Typedef for Structures and Unions ……………………………………………………………………………………… 237
Section 42.2: Typedef for Function Pointers ……………………………………………………………………………………………. 238
Section 42.3: Simple Uses of Typedef ……………………………………………………………………………………………………… 239
Chapter 43: Storage Classes ……………………………………………………………………………………………………………… 241
Section 43.1: auto ……………………………………………………………………………………………………………………………………. 241
Section 43.2: register ……………………………………………………………………………………………………………………………… 241
Section 43.3: static ………………………………………………………………………………………………………………………………….. 242
Section 43.4: typedef ……………………………………………………………………………………………………………………………… 243
Section 43.5: extern ………………………………………………………………………………………………………………………………… 243
Section 43.6: _Thread_local …………………………………………………………………………………………………………………… 244
Chapter 44: Declarations …………………………………………………………………………………………………………………… 246
Section 44.1: Calling a function from another C file ………………………………………………………………………………….. 246
Section 44.2: Using a Global Variable ……………………………………………………………………………………………………… 247
Section 44.3: Introduction ……………………………………………………………………………………………………………………….. 247
Section 44.4: Typedef …………………………………………………………………………………………………………………………….. 250
Section 44.5: Using Global Constants ……………………………………………………………………………………………………… 250
Section 44.6: Using the right-left or spiral rule to decipher C declaration …………………………………………………. 252
Chapter 45: Structure Padding and Packing ………………………………………………………………………………… 256
Section 45.1: Packing structures ……………………………………………………………………………………………………………… 256
Section 45.2: Structure padding ……………………………………………………………………………………………………………… 257
Chapter 46: Memory management …………………………………………………………………………………………………. 258
Section 46.1: Allocating Memory ……………………………………………………………………………………………………………… 258
Section 46.2: Freeing Memory ………………………………………………………………………………………………………………… 259
Section 46.3: Reallocating Memory …………………………………………………………………………………………………………. 261
Section 46.4: realloc(ptr, 0) is not equivalent to free(ptr) …………………………………………………………………………. 262
Section 46.5: Multidimensional arrays of variable size …………………………………………………………………………….. 262
Section 46.6: alloca: allocate memory on stack ………………………………………………………………………………………. 263
Section 46.7: User-defined memory management ………………………………………………………………………………….. 264
Chapter 47: Implementation-defined behaviour …………………………………………………………………………. 266
Section 47.1: Right shift of a negative integer ………………………………………………………………………………………….. 266
Section 47.2: Assigning an out-of-range value to an integer ……………………………………………………………………. 266
Section 47.3: Allocating zero bytes ………………………………………………………………………………………………………….. 266
Section 47.4: Representation of signed integers ……………………………………………………………………………………… 266
Chapter 48: Atomics …………………………………………………………………………………………………………………………….. 267
Section 48.1: atomics and operators ……………………………………………………………………………………………………….. 267
Chapter 49: Jump Statements ………………………………………………………………………………………………………….. 268
Section 49.1: Using return ……………………………………………………………………………………………………………………….. 268
Section 49.2: Using goto to jump out of nested loops ……………………………………………………………………………… 268
Section 49.3: Using break and continue ………………………………………………………………………………………………….. 269
Chapter 50: Create and include header files ………………………………………………………………………………… 271
Section 50.1: Introduction ……………………………………………………………………………………………………………………….. 271
Section 50.2: Self-containment ……………………………………………………………………………………………………………….. 271
Section 50.3: Minimality ………………………………………………………………………………………………………………………….. 273
Section 50.4: Notation and Miscellany …………………………………………………………………………………………………….. 273
Section 50.5: Idempotence ……………………………………………………………………………………………………………………… 275
Section 50.6: Include What You Use (IWYU) …………………………………………………………………………………………….. 275
Chapter 51: — character classification & conversion ………………………………………………. 277
Section 51.1: Introduction ………………………………………………………………………………………………………………………… 277
Section 51.2: Classifying characters read from a stream …………………………………………………………………………. 278
Section 51.3: Classifying characters from a string ……………………………………………………………………………………. 279
Chapter 52: Side Eects ……………………………………………………………………………………………………………………… 280
Section 52.1: Pre/Post Increment/Decrement operators …………………………………………………………………………. 280
Chapter 53: Multi-Character Character Sequence ………………………………………………………………………. 282
Section 53.1: Trigraphs ……………………………………………………………………………………………………………………………. 282
Section 53.2: Digraphs ……………………………………………………………………………………………………………………………. 282
Chapter 54: Constraints ………………………………………………………………………………………………………………………. 284
Section 54.1: Duplicate variable names in the same scope ………………………………………………………………………. 284
Section 54.2: Unary arithmetic operators ……………………………………………………………………………………………….. 284
Chapter 55: Inlining ………………………………………………………………………………………………………………………………. 285
Section 55.1: Inlining functions used in more than one source file …………………………………………………………….. 285
Chapter 56: Unions ………………………………………………………………………………………………………………………………… 287
Section 56.1: Using unions to reinterpret values ………………………………………………………………………………………. 287
Section 56.2: Writing to one union member and reading from another ……………………………………………………. 287
Section 56.3: Dierence between struct and union ………………………………………………………………………………….. 288
Chapter 57: Threads (native) …………………………………………………………………………………………………………….. 289
Section 57.1: Inititialization by one thread ………………………………………………………………………………………………… 289
Section 57.2: Start several threads ………………………………………………………………………………………………………….. 289
Chapter 58: Multithreading ………………………………………………………………………………………………………………… 291
Section 58.1: C11 Threads simple example ……………………………………………………………………………………………….. 291
Chapter 59: Interprocess Communication (IPC) ……………………………………………………………………………. 292
Section 59.1: Semaphores ……………………………………………………………………………………………………………………….. 292
Chapter 60: Testing frameworks ……………………………………………………………………………………………………… 297
Section 60.1: Unity Test Framework ………………………………………………………………………………………………………… 297
Section 60.2: CMocka ……………………………………………………………………………………………………………………………… 297
Section 60.3: CppUTest …………………………………………………………………………………………………………………………… 298
Chapter 61: Valgrind ……………………………………………………………………………………………………………………………… 300
Section 61.1: Bytes lost — Forgetting to free …………………………………………………………………………………………….. 300
Section 61.2: Most common errors encountered while using Valgrind ………………………………………………………. 300
Section 61.3: Running Valgrind ………………………………………………………………………………………………………………… 301
Section 61.4: Adding flags ……………………………………………………………………………………………………………………….. 301
Chapter 62: Common C programming idioms and developer practices ………………………………. 302
Section 62.1: Comparing literal and variable ……………………………………………………………………………………………. 302
Section 62.2: Do not leave the parameter list of a function blank — use void …………………………………………… 302
Chapter 63: Common pitfalls ……………………………………………………………………………………………………………… 305
Section 63.1: Mixing signed and unsigned integers in arithmetic operations ……………………………………………… 305
Section 63.2: Macros are simple string replacements ……………………………………………………………………………… 305
Section 63.3: Forgetting to copy the return value of realloc into a temporary ………………………………………….. 307
Section 63.4: Forgetting to allocate one extra byte for \0 ……………………………………………………………………….. 308
Section 63.5: Misunderstanding array decay …………………………………………………………………………………………… 308
Section 63.6: Forgetting to free memory (memory leaks) ……………………………………………………………………….. 310
Section 63.7: Copying too much ……………………………………………………………………………………………………………… 311
Section 63.8: Mistakenly writing = instead of == when comparing …………………………………………………………….. 312
Section 63.9: Newline character is not consumed in typical scanf() call …………………………………………………… 313
Section 63.10: Adding a semicolon to a #define ………………………………………………………………………………………. 314
Section 63.11: Incautious use of semicolons ……………………………………………………………………………………………… 314
Section 63.12: Undefined reference errors when linking …………………………………………………………………………… 315
Section 63.13: Checking logical expression against ‘true’ ………………………………………………………………………….. 317
Section 63.14: Doing extra scaling in pointer arithmetic …………………………………………………………………………… 318
Section 63.15: Multi-line comments cannot be nested ………………………………………………………………………………. 319
Section 63.16: Ignoring return values of library functions …………………………………………………………………………. 321
Section 63.17: Comparing floating point numbers ……………………………………………………………………………………. 321
Section 63.18: Floating point literals are of type double by default …………………………………………………………… 323
Section 63.19: Using character constants instead of string literals, and vice versa ……………………………………. 323
Section 63.20: Recursive function — missing out the base condition ………………………………………………………… 324
Section 63.21: Overstepping array boundaries ………………………………………………………………………………………… 325
Section 63.22: Passing unadjacent arrays to functions expecting “real” multidimensional arrays …………….. 326
Credits ………………………………………………………………………………………………………………………………………………………. 328
You may also like …………………………………………………………………………………………………………………………………… 333
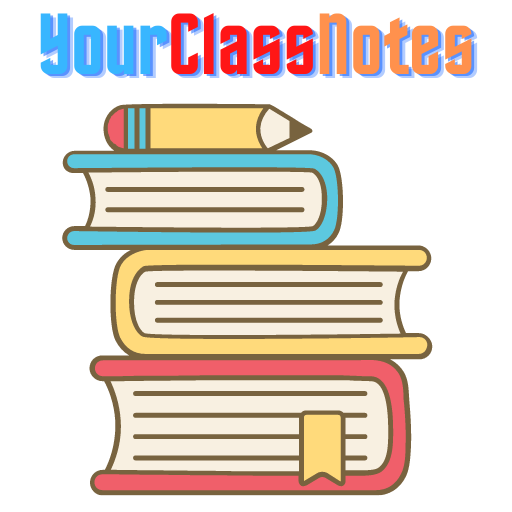
The person behind this site is an NIT (National Institute of Technology) Grad with 20+ years of industry experience. The team has nicknamed him Mr. ClassNotes. With a group of blogs and websites, Mr. ClassNotes shares his knowledge and experience with global readers.